【A学习】Homework-of-C-Language
概述:3gstudent/Homework-of-C-Language: C/C++ code examples of my blog. 仓库学习记录
[toc]
0x01 CheckCriticalProess
说明: 检查进程是不是关键进程
相关链接:
windows关键进程列表:
- 内核的系统进程(NTOSKrnl.exe)
- 会话管理器子系统(SMSS.exe)
- 客户端服务器运行时子系统(CSRSS.exe)
- Windows登录(WinLogon.exe)
- Windows Init(WinInit.exe)
- 仅适用于RDP的 Windows登录用户界面主机(LogonUI.exe)
- 本地安全机构进程(lsass.exe)
- 服务控制管理器(Services.exe)
- 使用RPCSS或Dcom / PnP的 服务主机(svchost.exe)
- 桌面窗口管理器(DWM.exe)
- 加上其他可选流程,如性能监控 或Internet Information Server(ISS)
步骤
- 获取进程 PID
- 通过 PID 打开进程
- 获取 ntdll.dll 中的导出函数
NtQueryInformationProcess
- 通过
NtQueryInformationProcess
获取ProcessBreakOnTermination
的值
代码说明
主要通过 ntdll 中的接口 NtQueryInformationProcess
获取 ProcessBreakOnTermination
的值。从 windows 8.1 开始可以使用 IsProcessCritical
来判断。
1 |
|
0x02 CheckUserBadPwdPolicy
说明:获取域内用户的 badPasswordTime 和 badPwdCount 属性
相关链接:
- ADsOpenObject 函数 (adshlp.h) - Win32 apps | Microsoft Learn
该函数用户绑定到 ADSI 对象,但如果传入的用户名和密码均为空,则会使用运行程序的用户账户上线文进行绑定。
关键代码
1 |
|
FileMapping
说明:创建文件映射以共享数据
CreateFileMapping
说明:创建文件映射
步骤
-
初始化安全描述符
SECURITY_ATTRIBUTES
,该安全描述符描述了子进程是否可以继承当前句柄。结构体如下所示:1
2
3
4
5typedef struct _SECURITY_ATTRIBUTES {
DWORD nLength;
LPVOID lpSecurityDescriptor;
BOOL bInheritHandle;
} SECURITY_ATTRIBUTES, *PSECURITY_ATTRIBUTES, *LPSECURITY_ATTRIBUTES;- nLength: 当前结构大小
- lpSecurityDescription: 指向
SECURITY_DESCRIPTOR
的指针,该结构保存了查询对象的安全状态。 - bInheritHandle: 创建新进程时是否继承当前句柄,默认为 FALSE
-
调用
CreateFileMapping
为指定文件创建或打开命名或未命名的文件映射对象。 -
调用
MapViewOfFile
,创建文件映射的视图到进程内存地址的映射,该函数会返回映射在进程空间的内存地址Address -
写入数据到步骤2返回的Address中
CreateFileMapping
创建"Global\*"
前缀的对象时,需要管理员权限。不需要管理员权限情况下可以使用Local\*
前缀来创建对象。
主要代码
创建安全描述符
1 |
|
创建文件映射到内存的映射,并写入数据
1 |
|
OpenFileMapping
说明:打开文件映射并读取文件映射中的数据。
步骤
与创建文件共享不同,打开并读取的步骤相对简单。
- 调用
OpenFileMapping
打开一个指定名称的文件共享对象 - 调用
MapViewOfFile
获取文件共享的地址 Address - 读取 Address 地址的内容
主要代码
1 |
|
CreateRemoteThread
主要就是在目标进程中调用 LoadLibrary
和 FreeLibrary
这两个函数。
- CreateRemoteThread
- LoadLibrary
- Freelibrary
- OpenProcess
- VirtualAllocEx
- GetProcAddress
- CreateToolhelp32Snapshot
注意点
卸载dll时需要判断dll是否存在。
需要调用 CreateToolhelp32Snapshot
通过 MODULEENTRY32
枚举进程加载的模块有哪些。MODULEENTRY32
结构体如下所示
- szModule:模块名
- szExePath:模块加载的路径
1 |
|
创建远程线程加载dll
1 |
|
创建远程线程卸载dll
1 |
|
DeleteRecordFileCache
RecentFileCahce.bcf 文件说明
RecentFileCache.bcf 文件是 win7 操作系统下特有的文件,用来跟踪应用程序与不同可执行文件的兼容性问题,能够记录应用程序执行的历史记录。 win8及以上的系统不支持该文件。
- 文件位置:
C:\Windows\AppCompat\Programs\RecentFileCache.bcf
可以用 winhex 打开查看文件内容,如下所示:
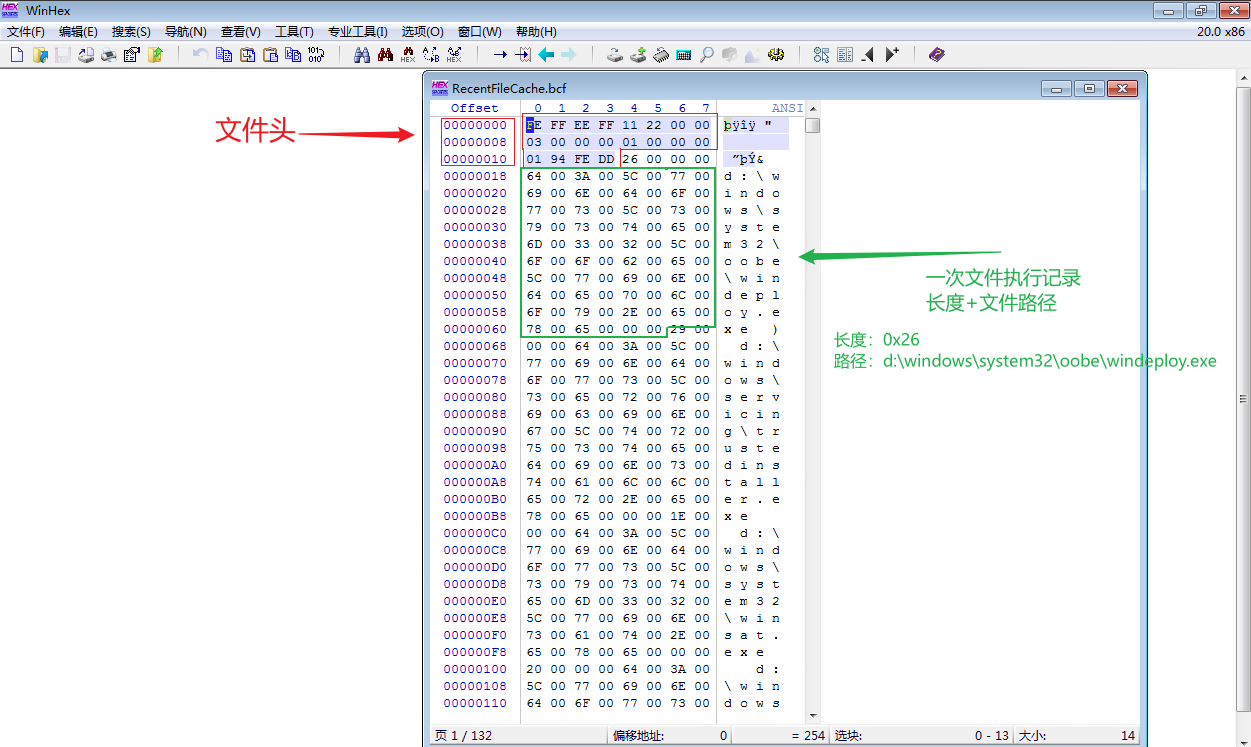
从图中可以看出 RecenFileCache 文件的结构,20字节的文件头,之后就是 4字节长度加文件执行路径这样的格式了。
根据文件结构定义了以下两个结构体
1 |
|
1 |
|
注:
ULONG64为8字节,ULONG为4字节
逐个解析每条记录,通过固定变量Size确定记录长度,进而读取每条记录的内容
读取 RecentFileCache.bcf 文件。
读取 RecentFileCache 文件时,需要注意文件头部分,文件头出的 shellcode 是固定的。
ShellCode 说明
RecentFileCache.bcf 固定的文件头内容
1 |
|
代码
1 |
|
删除记录
删除记录基本同读取逻辑一致。由于 RecentFileCache 是按一定的结构写入的,因此在删除时也需要按相应的结构操作。
即:删除一条记录=删除记录长度和记录的内容
代码
1 |
|
MasqueradePEBToCopyfile
进程伪装成 explorer.exe 进而达到进程提权的目的
参考代码:hfiref0x/UACME at 143ead4db6b57a84478c9883023fbe5d64ac277b
UAC触发流程
在触发 UAC
时,系统会创建一个consent.exe
进程,该进程用以确定是否创建管理员进程(通过白名单和用户选择判断),然后creatprocess
请求进程,将要请求的进程cmdline和进程路径通过LPC接口传递给appinfo的RAiLuanchAdminProcess函数,该函数首先验证路径是否在白名单中,并将结果传递给consent.exe进程,该进程验证被请求的进程签名以及发起者的权限是否符合要求,然后决定是否弹出UAC框让用户进行确认。这个UAC框会创建新的安全桌面,屏蔽之前的界面。同时这个UAC框进程是SYSTEM权限进程,其他普通进程也无法和其进行通信交互。用户确认之后,会调用CreateProcessAsUser函数以管理员权限启动请求的进程
调用说明
修改参数:
接下来需要添加修改PEB结构的功能,为了欺骗PSAPI,共需要修改以下位置:
-
_RTL_USER_PROCESS_PARAMETERS.ImagePathName
-
_RTL_USER_PROCESS_PARAMETERS.CommandLine(可选)
-
_LDR_DATA_TABLE_ENTRY.FullDllName
-
_LDR_DATA_TABLE_ENTRY.BaseDllName
属性说明:
- FOF_NOCONFIRMATION :不弹出确认框
- FOF_SILENT:不弹框
- FOFX_SHOWELEVATIONPROMPT:需要提升权限
- FOFX_NOCOPYHOOKS:不使用copy hooks
- FOFX_REQUIREELEVATION:默认需要提升权限
- FOF_NOERRORUI:报错不弹框
区别
普通进程需要执行两步,伪装进程只需要第二步的提权
- 获取进程权限
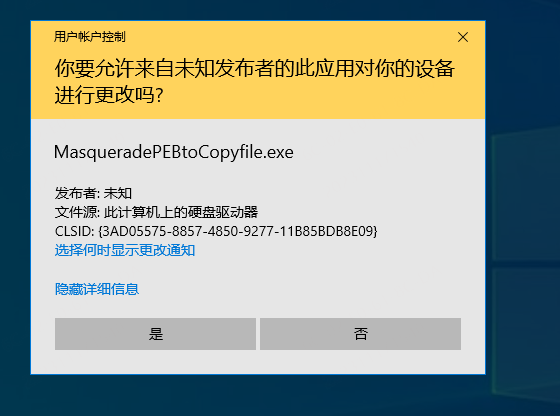
- 获取操作权限
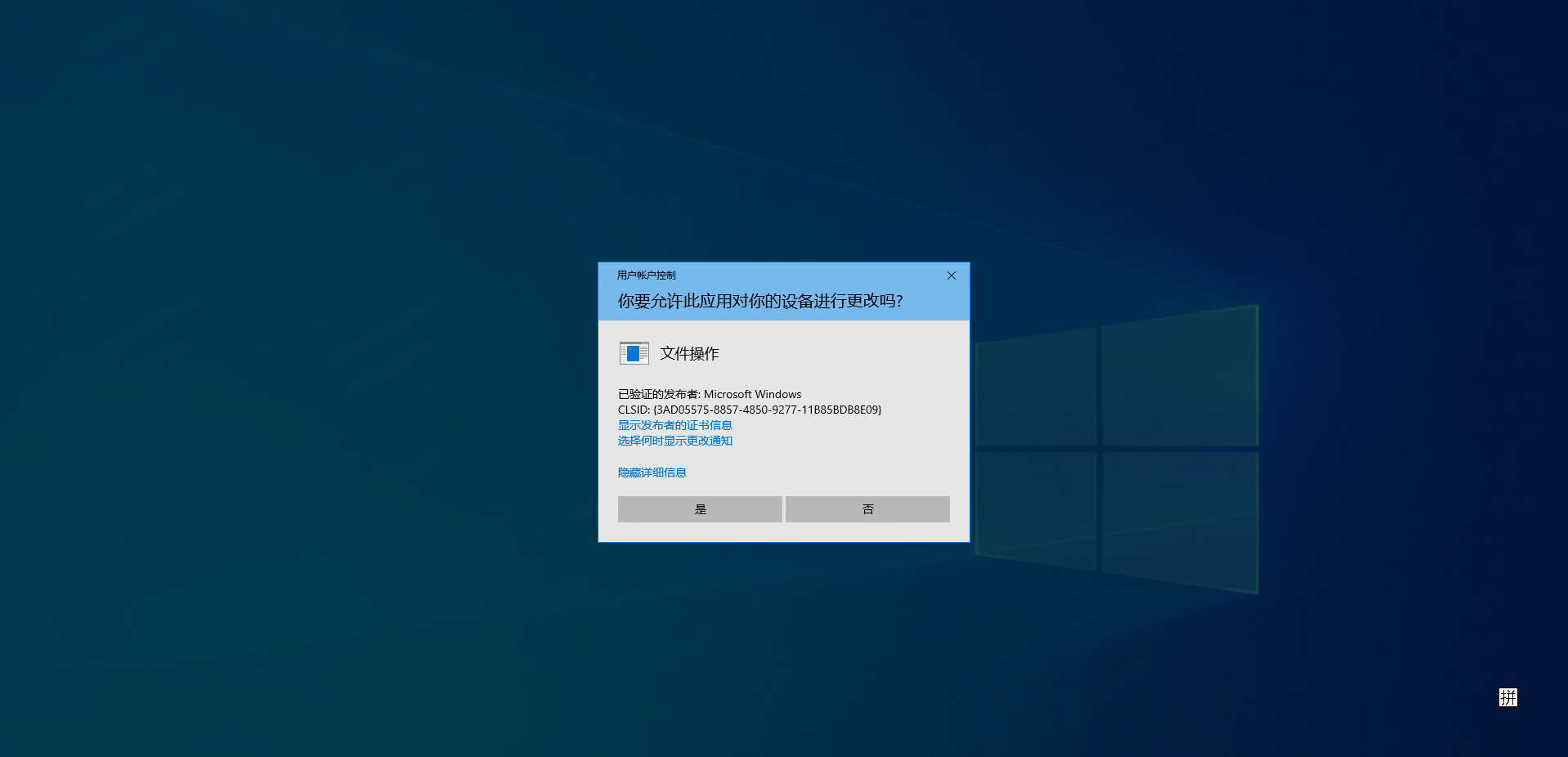
调用分析
堆栈
伪装进程调用栈,执行操作的提权最终调用的 combase!CComActivator::StandardCreateInstance
,函数原型见后文。但是普通进程在执行操作前需要调用 _CreateElevatedCopyengine
对当前进程先提权。
1 |
|
调用堆栈,由上而下
1 |
|
_RunElevatedOperation 汇编代码:
主要关注一下几个函数:
- windows_storage!CFileOperation::_GetElevatedOperation (7546f148)
- windows_storage!CFileOperation::_CreateElevatedCopyengine (7546e321)
- windows_storage!CFileOperation::_PerformProperElevatedOperations (7547155d)
1 |
|
ole32!CoGetObject:
1 |
|
普通进程
1 |
|
伪装进程
1 |
|
下面是CoGetObject的函数原型和可能的实现:
1 |
|
BindToObject 汇编代码:
1 |
|
CComActivator::StandardCreateInstance 定义:
1 |
|
DoCreateInstance 定义:
1 |
|
ICoCreateInstanceEx 定义:
1 |
|
combase!ScmRequestInfo::SetScmInfo 定义:
1 |
|
DeleteRecordbyTerminateProces(ReplaceFile)
概述:通过替换文件的方式删除系统日志文件
步骤
- 查找 eventlog 服务进程,获取 pid
- 获取进程句柄并关闭进程
- 拷贝临时日志文件到对应的路径
主要代码
获取 eventlog 服务进程
1 |
|
DisableFireWall
关闭防火墙策略
com 越权实现关闭防火墙策略。